基于eloquent orm的缓存实时更新、读取封装 | laravel china 社区-380玩彩网官网入口
基于eloquent orm的缓存实时更新、读取封装
一、设计背景:
有不少公司的后台系统在操作更新数据库的同时会实时刷新缓存(永久),而且缓存一般都采用hash(存表的行数据) 有序集合(指定排序字段存放行数据的id)。但是每个模型都加这些操作缓存的方法实在是繁琐的很,其实都大同小异。那我们有没有办法去设计一套规则去吃掉这些繁琐的操作呢?答案是肯定有的,接下来我会提出我的解决思路并用代码实现这个过程。我一直是秉着用最少的代码去实现同样的功能的原则去设计每一样东西,这样我们的coding质量才能得到大大的提高。
二、设计思路
只要我们能够监听到操作完数据库后,我们具体做了哪些操作(新增、更新、删除)并且能够拿到操作时对应的数据,我们就能对这些数据去做相应的缓存处理。都统一封装起来,作为模型基类去被其他模型类继承使用即可,同时考虑到一些比较特殊的情况,可能需要特殊处理的,在合适的位置开放方法让模型类实现一下即可。
laravel orm自带的就能监听并拿到数据。
所以我们只要在相应的事件中做相应的处理就好了。
三、coding time
1.这是我的模型基类(base.php),分别监听不同事件做了不同的缓存处理
namespace app\models;
use app\models\cache\basecache;
use app\models\cache\querycache;
use app\models\cache\relationcache;
use illuminate\support\facades\cache;
use illuminate\database\eloquent\model;
class base extends model
{
protected $guarded = [];
public $timestamps = false;
/**
* @var null
* 缓存实例
*/
protected static $cache = null;
/**
* @var bool
* orm缓存开关
*/
protected static $cacheswitch = false;
use basecache,relationcache,querycache;
public function __construct(array $data = [])
{
parent::__construct($data);
$this->initcache();
}
protected static function booted()
{
parent::booted(); // todo: change the autogenerated stub
if (static::$cacheswitch) {
$calledclass = get_called_class();
static::saved(function ($saved) use ($calledclass){
$original = $saved->original;
if (empty($original)) {
var_dump('created');
//新增缓存
$createdbaseres = static::createdbase($saved);
$createdrelationres = true;
if (static::$relationcachekey) {
$createdrelationres = static::createdrelation($saved);
}
//更新缓存后的回调处理
if (method_exists($calledclass, 'createdcachecallback')) {
static::createdcachecallback($saved, $createdbaseres && $createdrelationres);
}
} else {
var_dump('updated');
//更新缓存
$updatedbaseres = static::updatedbase($saved);
$updatedrelationres = true;
if (static::$relationcachekey) {
$updatedrelationres = static::updatedrelation($saved);
}
if (method_exists($calledclass, 'updatedcachecallback')) {
static::updatedcachecallback($saved, $updatedbaseres && $updatedrelationres);
}
}
});
static::deleted(function ($deleted) use ($calledclass){
var_dump('deleted');
//删除缓存
$deletedbaseres = static::deletedbase($deleted);
$deletedrelationres = true;
if (static::$relationcachekey) {
$deletedrelationres = static::deletedrelation($deleted);
}
if (method_exists($calledclass, 'deletedcachecallback')) {
static::deletedcachecallback($deleted, $deletedbaseres && $deletedrelationres);
}
});
}
}
/**
* @param array $data
* @param string $cachekey
* @return string
* @throws \exception
* 获取缓存key
*/
public static function getcachekey(array $data, string $cachekey, bool $rewritedata = false): string
{
$pattern = '/{\$(.*?)}/';
preg_match_all($pattern, $cachekey, $matches);
$variables = $matches[1];
$find = $replace = [];
if ($rewritedata) {
foreach ($variables as $vk => $vv) {
if (isset($data[$vk])) {
$data[$vv] = $data[$vk];
}
}
}
foreach ($variables as $variable) {
if (!isset($data[$variable])) {
throw new \exception("获取缓存key失败:缺失{\${$variable}}");
}
$find[] = "{\$$variable}";
$replace[] = $data[$variable];
}
return $find && $replace ? str_replace($find,$replace,$cachekey) : $cachekey;
}
/**
* @param array $data
* @return void
* 过滤缓存字段
*/
public static function filtercachedatafield(array &$data)
{
if (static::$cachefields) {
foreach ($data as $k => $v) {
if(!in_array($data, static::$cachefields)) {
unset($data[$k]);
}
}
}
}
/**
* @param bool $paging
* @return void
* 开启分页
*/
public function setpaging(bool $paging)
{
static::$paging = $paging;
}
/**
* @int $pagesize
* @return void
* 设置每页记录数
*/
public function setpagesize(int $pagesize)
{
static::$pagesize = $pagesize;
}
/**
* @int $pagesize
* @return void
* 设置当前页码
*/
public function setpage(int $page)
{
static::$page = $page;
}
/**
* @param array $fields
* @return void
* 设置获取字段
*/
public function setgetfields(array $fields)
{
static::$getfields = $fields;
}
/**
* @param string $field
* @return void
* 设置排序字段
*/
public function setsortfield(string $field)
{
static::$sortfield = $field;
}
/**
* @param string $type
* @return void
* 设置排序顺序
*/
public function setsorttype(string $type)
{
static::$sorttype = $type;
}
/**
* @return null
* 初始化缓存实例
*/
protected function initcache()
{
if (self::$cache == null) {
self::$cache = cache::store('redis')->getredis();
}
return self::$cache;
}
}
2.这是处理表行数据的缓存类basecache.php
namespace app\models\cache;
use app\models\base;
trait basecache
{
/**
* @var string 缓存key
*/
protected static $cachekey = '';
/**
* @var array 缓存字段 空数组则缓存表所有字段
*/
protected static $cachefields = [];
/**
* @var string 缓存的数据结构 默认哈希
*/
protected static $cachedatastructure = 'hash';
/**
* @param $created
* @return bool
* @throws \exception
* orm设置缓存数据
*/
public static function createdbase ($created): bool
{
self::checkbasecacheconf();
$cache = base::initcache();
$data = $created->attributes;
$key = base::getcachekey($data,static::$cachekey);
base::filtercachedatafield($data);
$cachemethod = 'hmset';
if (static::$cachedatastructure == 'string') {
$cachemethod = 'set';
$data = json_encode($data,json_unescaped_unicode);
}
return $cache->$cachemethod($key,$data);
}
/**
* @param $updated
* @return bool
* @throws \exception
* orm更新缓存数据
*/
public static function updatedbase ($updated): bool
{
self::checkbasecacheconf();
$cache = base::initcache();
$data = $updated->attributes;
$key = base::getcachekey($data,static::$cachekey);
base::filtercachedatafield($data);
$cachemethod = 'hmset';
if (static::$cachedatastructure == 'string') {
$cachemethod = 'set';
$olddata = json_decode($cache->get($key),true) ?: [];
$data = array_merge($olddata,$data);
$data = json_encode($data,json_unescaped_unicode);
}
return $cache->$cachemethod($key,$data);
}
/**
* @param $deleted
* @return bool
* orm删除缓存数据
*/
public static function deletedbase ($deleted): bool
{
self::checkbasecacheconf();
$cache = base::initcache();
$data = $deleted->attributes;
$key = base::getcachekey($data,static::$cachekey);
return $cache->del($key);
}
/**
* @return bool
* @throws \exception
* 校验参数配置
*/
private static function checkbasecacheconf(): bool
{
if (empty(static::$cachekey)) {
throw new \exception('缓存错误:缓存key必填');
}
if (!in_array(static::$cachedatastructure, ['hash','string'])) {
throw new \exception('缓存的数据结构错误:暂时只支持string和hash');
}
return true;
}
}
3.这是处理关联表数据的缓存类relationcache.php
namespace app\models\cache;
use app\models\base;
trait relationcache
{
/**
* @var string 关联缓存key 为空则不开启关联缓存
*/
protected static $relationcachekey = '';
/**
* @var string 关联缓存的数据结构 默认有序集合
*/
protected static $relationcachedatastructure = 'zset';
/**
* @var array 关联缓存排序字段 数据结构为zset时必填
*/
protected static $relationcachesortfields = [];
/**
* @param $created
* @return bool
* @throws \exception
* orm设置缓存数据
*/
public static function createdrelation ($created): bool
{
$data = $created->attributes;
$primarykey = $created->primarykey;
if (!isset($data[$primarykey])) {
throw new \exception('关联缓存错误:主键数据不能为空');
}
self::checkrelationcacheconf();
$cache = base::initcache();
$cachekey = base::getcachekey($data,static::$relationcachekey);
$keys = static::$relationcachedatastructure == 'zset' ? self::getsortedkeys($cachekey) : [];
$res = true;
if ($keys) {
//开启事务
$cache->multi();
foreach ($keys as $kk => $kv) {
if (isset($data[$kk])) {
$cache->zadd($kv, $data[$kk], $data[$primarykey]);
}
}
//执行事务
$exec = $cache->exec();
foreach ($exec as $v) {
if($v === false){
$res = false;
break;
}
}
} else {
$res = $cache->sadd($cachekey, $data[$primarykey]);
}
return $res;
}
/**
* @param $updated
* @return bool
* @throws \exception
* orm更新缓存数据
*/
public static function updatedrelation ($updated): bool
{
self::checkrelationcacheconf();
//如果是有序集合
$res = true;
if (static::$relationcachedatastructure == 'zset') {
$cache = base::initcache();
$data = $updated->attributes;
$original = $updated->original;
$diff = array_diff_assoc($data,$original);
$intercept = array_intersect(array_keys($data), static::$relationcachesortfields);
if ($intercept && $diff) {
$primarykey = $updated->primarykey;
$cachekey = base::getcachekey($original,static::$relationcachekey);
$keys = self::getsortedkeys($cachekey);
$cache->multi();
foreach ($keys as $kk => $kv) {
if (isset($diff[$kk])) {
$cache->zadd($kv, $diff[$kk], $original[$primarykey]);
}
}
//执行事务
$exec = $cache->exec();
foreach ($exec as $v) {
if($v === false){
$res = false;
break;
}
}
}
}
return $res;
}
/**
* @param $deleted
* @return bool
* @throws \exception
* orm删除缓存数据
*/
public static function deletedrelation ($deleted): bool
{
self::checkrelationcacheconf();
$cache = base::initcache();
$res = true;
$original = $deleted->original;
$primarykey = $deleted->primarykey;
$cachekey = base::getcachekey($original,static::$relationcachekey);
if (static::$relationcachedatastructure == 'zset') {
$keys = self::getsortedkeys($cachekey);
$cache->multi();
foreach ($keys as $kk => $kv) {
$cache->zrem($kv, $original[$kk], $original[$primarykey]);
}
//执行事务
$exec = $cache->exec();
foreach ($exec as $v) {
if($v === false){
$res = false;
break;
}
}
} else {
$res = $cache->srem($cachekey,$original[$primarykey]);
}
return $res;
}
/**
* @param $key
* @return array
* @throws \exception
* 获取有序集合排序字段对应的key
*/
private static function getsortedkeys($key): array
{
$keys = [];
foreach (static::$relationcachesortfields as $sortfield) {
$keys[$sortfield] = "{$sortfield}_{$key}";
}
return $keys;
}
/**
* @return bool
* @throws \exception
* 检验参数配置
*/
private static function checkrelationcacheconf (): bool
{
if (!in_array(static::$relationcachedatastructure, ['zset','set'])) {
throw new \exception('关联缓存错误:数据结构错误,暂时只支持zset和set');
}
if (static::$relationcachedatastructure == 'zset' && empty(static::$relationcachesortfields)) {
throw new \exception('关联缓存错误:数据结构为zset时排序字段必填');
}
return true;
}
}
4.querycache.php提供缓存查询详情、列表(支持分页、排序设置)
namespace app\models\cache;
use app\models\base;
trait querycache
{
/**
* @var bool
* 开启分页
*/
protected static $paging = false;
/**
* @var int
* 设置当前页码
*/
protected static $page = 1;
/**
* @var int
* 设置每页记录数
*/
protected static $pagesize = 10;
/**
* @var array
* 获取的字段 为空默认返回所有
*/
protected static $getfields = [];
/**
* @var string
* 排序字段
*/
protected static $sortfield = '';
/**
* @var string
* 排序方式
*/
protected static $sorttype = 'desc';
/**
* @param ...$args
* @return array|mixed
* @throws \exception
* 获取缓存详情 按缓存key的{$xxx}顺序传参即可
*/
public function getinfo(...$args)
{
$cache = base::initcache();
$cachekey = base::getcachekey($args,static::$cachekey,true);
if (static::$cachedatastructure == 'string') {
$info = json_decode($cache->get($cachekey),true) ?? [];
} else {
$info = static::$getfields ? $cache->hmget($cachekey,static::$getfields) : $cache->hgetall($cachekey);
}
return $info;
}
/**
* @param ...$args
* @return array
* @throws \exception
* 获取缓存列表 按关联缓存key的{$xxx}顺序传参即可
*/
public function getlist(...$args)
{
$cahce = base::initcache();
$relationcachekey = base::getcachekey($args,static::$relationcachekey,true);
$list = [];
if (static::$relationcachedatastructure == 'zset') {
$key = static::$sortfield ? static::$sortfield.'_'.$relationcachekey : static::$relationcachesortfields[0].'_'.$relationcachekey;
$start = 0;
$end = -1;
if (static::$paging) {
//开启分页
$start = (static::$page - 1) * static::$pagesize;
$end = $start static::$pagesize - 1;
}
$elements = strtolower(static::$sorttype) == 'asc' ? $cahce->zrange($key, $start, $end) : $cahce->zrevrange($key, $start, $end);
} else {
$elements = [];
if (static::$paging) {
//开启分页
$start = (static::$page - 1) * static::$pagesize;
$end = $start static::$pagesize - 1;
// 使用 sscan 迭代获取指定范围内的元素
$cursor = '0'; // 初始游标值
do {
$result = $cahce->sscan($relationcachekey, $cursor, 'match', '*', 'count', static::$pagesize);
$cursor = $result[0]; // 获取下一次迭代的游标值
$elements = array_merge($elements, $result[1]); // 将获取到的元素合并到结果数组中
} while ($cursor !== '0' && count($elements) < $end); // 继续迭代直到游标为 '0' 或达到结束索引
// 截取指定范围内的元素
$elements = array_slice($elements, $start, static::$pagesize);
} else {
$elements = $cahce->smembers($relationcachekey);
}
}
foreach ($elements as $member) {
$list[] = self::getinfo($member);
}
return $list;
}
}
四、demo测试
1.建个模型admuser.php继承base.php模型
namespace app\models;
class admuser extends base
{
protected $table = 'adm_user';
protected static $cacheswitch = true;
protected static $cachekey = 'au:{$id}';
protected static $relationcachekey = 'aur';
protected static $relationcachesortfields = ['id','buy', 'view'];
/**
* @param $created
* @return void
* orm设置缓存回调处理
*/
protected static function createdcachecallback($created, $cacheres){
var_dump($cacheres);
}
/**
* @param $updated
* @return void
* orm更新缓存回调处理
*/
protected static function updatedcachecallback($updated, $cacheres){
var_dump($cacheres);
}
/**
* @param $deleted
* @return void
* orm删除缓存回调处理
*/
protected static function deletedcachecallback($deleted, $cacheres){
var_dump($cacheres);
}
}
2.建个控制器indexcontroller.php 分开执行cud、查看缓存更新情况!
namespace app\http\controllers\web;
use app\models\admuser;
use laravel\lumen\routing\controller;
class indexcontroller extends controller
{
public function index()
{
//模拟插入数据
for ($i = 0; $i < 30; $i) {
(admuser::query()->create([
'name'=>uniqid(),
'age'=>mt_rand(18,50),
'buy'=>mt_rand(0,3000),
'view'=>mt_rand(0,30000),
'created_at'=>time()
]));
}
//查询缓存数据
//设置返回字段
// admuser::setgetfields(['age']);
//查询详情
$info = admuser::getinfo(1);
var_dump($info);
//查询列表 开启分页
admuser::setpaging(true);
//设置当前页码
admuser::setpage(1);
//设置每页记录数
admuser::setpagesize(5);
//设置排序字段
admuser::setsortfield('view');
//设置排序方式
admuser::setsorttype('asc');
$list = admuser::getlist();
var_dump($list);
//更新数据
$au = admuser::find(1);
$au->name = 123456;
$au->age = 11;
$au->buy = 22;
$au->created_at = time();
$au->save();
//删除数据
admuser::destroy([1]);
}
}
3.这是我本地跑的一些数据
以上就是这次的orm缓存实时更新设计、后期有新想法还可以拓展自己想要的东西!!!谢谢观看!!!
本作品采用《cc 协议》,转载必须注明作者和本文链接
过度封装
想法很棒,建议看下
这里是不是写错了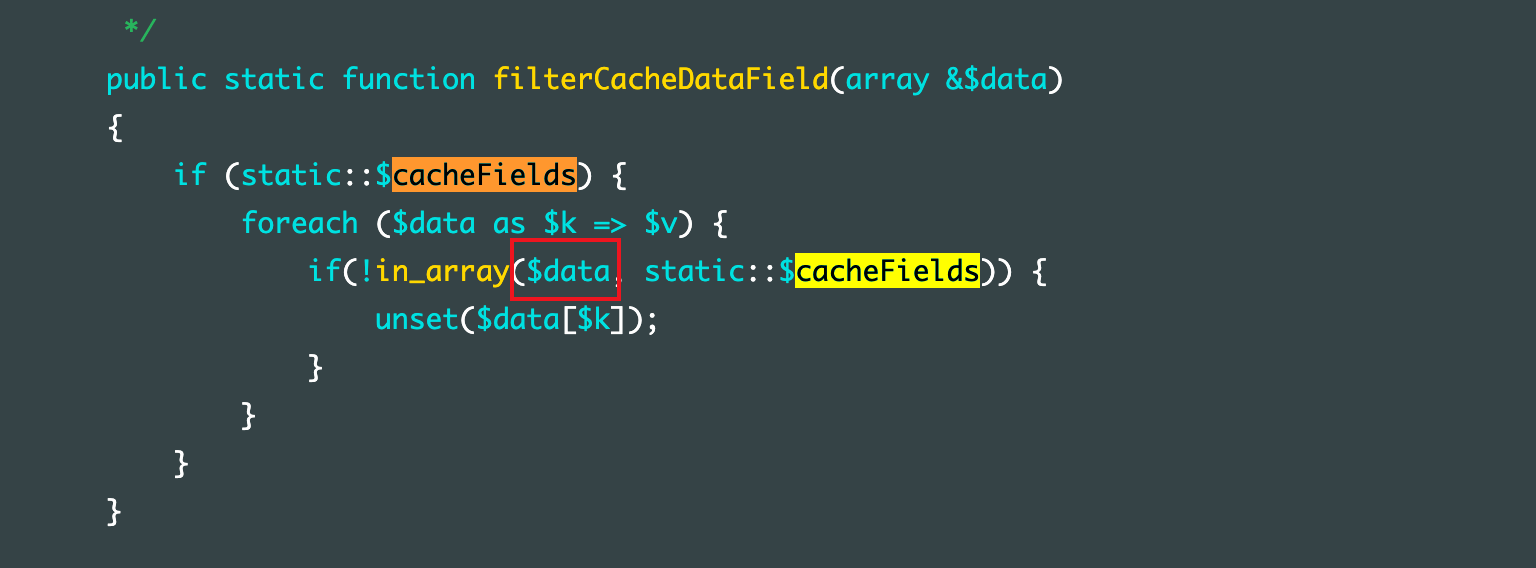